Config Validation & Signing
The router configuration, updatable via CDN or file, employs a “Config Validation & Signing” feature to safeguard against tampering through external validation and signing.
Available since version 0.74.0
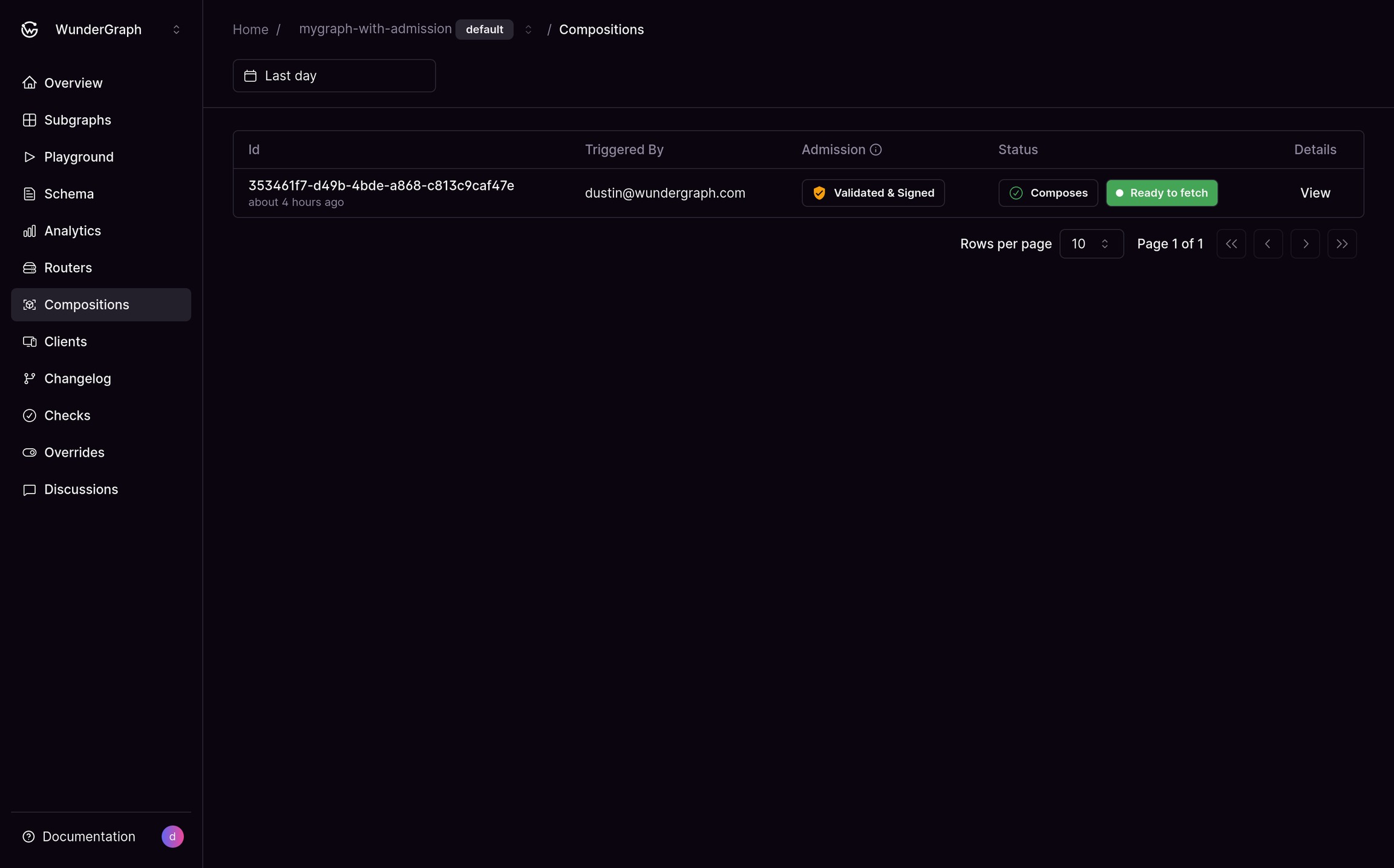
Validated & Signed composition
The router configuration includes a Graph Plan, which encapsulates all the necessary information for planning and executing queries. It also specifies the URLs of your subgraphs. This configuration can be periodically fetched from the CDN or downloaded via wgc
cli to the file system. In either scenario, the content originates from our platform. It is crucial to detect and mitigate tampering attacks, where an adversary might alter the configuration to reroute your traffic to an unauthorized server. To address this concern, we have developed a feature named “Config Validation & Signing” to identify and prevent such attacks.
When setting up a federated graph, you must use the --admission-webhook-url
option, pointing it to your publicly accessible admission server. Example: https://admission.example.com
(without the /validate-config
path name).
For security reasons, ensure your admission server uses HTTPS as transport prototcol.
Our system will invoke the /validate-config
handler on your server each time a composition occurs. Only in case of a successful composition and proper response of your admission hook the config is made available to your router.
The payload for this operation will be structured as follows:
It is your responsibility to retrieve the router configuration by making a GET request to the privateConfigUrl
validate the configuration, and then return a HMAC-SHA256 of the configuration, encoded in BASE64. The privateConfigUrl
contains a token that is short-lived (5min).
Once validation is successful, the configuration will no longer be availabe at the above URL and will return a 404
status code.
Webhook signature verification serves the same purpose as the signature verification of the router config. We want to ensure that the router config has not been tampered with after delivery. This procedure is very common today, almost all major webhook providers use the SHA-256 hash function in the SHA-2 series for signature verification of their webhooks. These webhook providers include Stripe, GitHub and Okta.
After you have calculated the hash, the expected response must have a 200 status code and contain the HMAC-SHA256 signature:
Alternatively, you can return a 400 status code with an error property to signal a validation error:
This will prevent the controlplane to deploy the composition. The error will be visible on the composition detail page in the Studio.
Sign Incoming Requests
To ensure that requests made to your admission webhook url are intended to you. You can set a secret using the --admission-webhook-secret
option when creating your graph. For existing graphs, the update command also allows you to set it. You need to compute the HMAC signature on your server and compare it to the signature in the X-Cosmo-Signature-256
header. Below is an example in Node.js
Router configuration
Upon receiving the signature, we will associate it with the artifact. The subsequent step involves initializing your router with the same signature key used during the hashing process on the admission server.
Example configuration:
The router will then calculate the artifact’s hash locally and compare it to the signature received from the CDN or filesystem. If the two match, the configuration is applied; otherwise, the router rejects it and terminates. If the router was already running successfully, it will continue to operate but will refuse to apply configurations that do not match the expected signature.
Instead of polling for updates from the CDN, the Graph Plan can also be passed via a file to the router. To leverage the same validation and signing mechanism in this approach, you must supply the --graph-sign-key
parameter to the wgc router fetch
command as well. This ensures consistency in security measures, whether the configuration is obtained from the CDN or directly from a file.
Config Validation
An attacker has various methods to influence the router’s behavior. Depending on their intent, they might aim to cause downtime or steal data. From an economic perspective, it is more likely that an attacker seeks to steal sensitive information. Therefore, we will focus on sections detailing how to validate the most important configuration settings.
Scenario - Manipulation of subgraph datasource URL’s
Description:
An attacker change the subgraph URL to redirect customer traffic to a different server.
Impact:
High (Possible outage with data leak)
Action:
Implement a check that ensures all subgraph URLs belong to the same domain of your organization. If not, return a non-200 status code with a descriptive error message. The error message will be visible in the studio. The exact check depends on your service configuration. If your subgraphs don’t belong to the same domain, you can maintain an allowlist and compare it with all the urls we encounter in the config.
We plan to encapsulate the most common validation rules into an NPM package, so you don’t have to deal with the internal configuration structure. For now, please take inspiration from the examples and seek for help when things are not clear.
Example Server Implementation
The admission webhook handler has to be publicly available. We provide an example implementation In Hono, a framework that can be deployed to multiple platform like Cloudflare Worker, Fastly, Deno, Bun or Node.js.
Was this page helpful?